C# ile yazılmış photoshop uygulaması türkçeleştirildi.
İngilizce;
Kod:
namespace ***Redacted****
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
//If the Load Button is clicked
private **** loadb_Click(object sender, EventArgs e)
{
//Open the file dialog and allow the user to pick an image
if (openFileDialog1.ShowDialog() == DialogResult.OK)
{
//Try to set the file they chose as the image in the picture box
try
{
pbox.Image******** = openFileDialog1.FileName;
//Enable the Transform button if it works
tb.Enabled = true;
}
//If the image setting fails, disable the transform button
catch
{
tb.Enabled = false;
}
}
}
//If the Transform! button is clicked
private **** tb_Click(object sender, EventArgs e)
{
//Load the image in the picture box as a bitmap
Bitmap bmp = new Bitmap(pbox.Image);
//Determin how large to make the progress bar
progressBar1.Maximum = bmp.Height;
//If the contrast radio is checked
if (contb.Checked)
{
//Use nested loops to obtain coordinate of each pixel
for (int y = 0; y < bmp.Height; y++)
{
progressBar1.Value = y+1;
for (int x = 0; x < bmp.Width; x++)
{
//Get the color of the pixel
Color argb = bmp.GetPixel(x, y);
int R;
int G;
int B;
//Detect if the value is over 128 or not
if (argb.R > 128)
{
//Make sure that the value won't go over 255
if (argb.R + (trackbar.Value / 5) > 255)
{
R = 255;
}
else
{
//Change the R value to a higher one
R = argb.R + (trackbar.Value / 5);
}
}
else
{
//Make sure the value wont go under 0
if (argb.R - (trackbar.Value / 5) < 0)
{
R = 0;
}
else
{
//Change the R value to a lower one
R = argb.R - (trackbar.Value / 5);
}
}
//Repeat for green
if (argb.G > 128)
{
if (argb.G + (trackbar.Value / 5) > 255)
{
G = 255;
}
else
{
G = argb.G + (trackbar.Value / 5);
}
}
else
{
if (argb.G - (trackbar.Value / 5) < 0)
{
G = 0;
}
else
{
G = argb.G - (trackbar.Value / 5);
}
}
//Repeat for Blue
if (argb.B > 128)
{
if (argb.B + (trackbar.Value / 5) > 255)
{
B = 255;
}
else
{
B = argb.B + (trackbar.Value / 5);
}
}
else
{
if (argb.B - (trackbar.Value / 5) < 0)
{
B = 0;
}
else
{
B = argb.B - (trackbar.Value / 5);
}
}
//Change the color of the pixel to its new value
argb = Color.FromArgb(argb.A, R, G, ;
//Apply the new color to the pixel
bmp.SetPixel(x, y, argb);
}
}
}
//If the tint radio is checked
if (tintb.Checked)
{
//Int for green
int G;
//Int for blue
int R;
//Nested for loops for iterating through every pixel
for (int y = 0; y < bmp.Height; y++)
{
//Adjusting the progress bar based on how far along we are
progressBar1.Value = y + 1;
for (int x = 0; x < bmp.Width; x++)
{
//Obtain the current pixels color
Color argb = bmp.GetPixel(x, y);
//Initialize the values to zero
R = 0;
G = 0;
//Determin if the we want to tint red or green
if (trackbar.Value >= 50)
{
//Set R to the proper value
R = argb.R;
//Ensure that the Value of G will stay within bounds as we adjust
if (((trackbar.Value - 50) + argb.G) > 255)
{
G = 255;
}
else
{
//Adjust the green value based on how much tint we want
G = argb.G + (trackbar.Value - 50);
}
}
if (trackbar.Value < 50)
{
//Set G to the proper value
G = argb.G;
//Ensure that the Value of R will stay within bounds as we adjust
if ((50 - trackbar.Value + argb.R) > 255)
{
R = 255;
}
else
{
//Adjust the red value based on how much tint we want
R = argb.R + (50 - trackbar.Value);
}
}
//Change the color of the pixel to its new value
argb = Color.FromArgb(argb.A, R, G, argb.;
//Apply the new color to the pixel
bmp.SetPixel(x, y, argb);
}
}
}
//If the black/white radio is checked
if (bwb.Checked)
{
//Double used to average RGB
double ave;
//Ints used to set RGB at the end of calculations
int Ri;
int Gi;
int Bi;
//Doubles used in calculations so we dont round
double R;
double G;
double B;
//A percentage based on how much greyscale we chose
double p = (double)trackbar.Value / 100;
//Nested for loops used to iterate through each pixel
for (int y = 0; y < bmp.Height; y++)
{
//Adjust the progress bar
progressBar1.Value = y + 1;
for (int x = 0; x < bmp.Width; x++)
{
//Get the color values of each pixel
Color argb = bmp.GetPixel(x, y);
//Assign the values to the respective double
R = argb.R;
G = argb.G;
B = argb.B;
//Determin the average
ave = (R + G + / 3;
//Cast the calculated black and white values to integers
Ri = (int)(R + ((ave - R) * p));
Gi = (int)(G + ((ave - G) * p));
Bi = (int)(B + ((ave - * p));
//Change the color of the pixel to its new value
argb = Color.FromArgb(argb.A, Ri, Gi, Bi);
//Apply the new color to the pixel
bmp.SetPixel(x, y, argb);
}
}
}
//If the noise radio is checked
if (nb.Checked)
{
//A random number to be used to generate noise
Random rnd = new Random();
//Red value
int R;
//Green value
int G;
//Blue value
int B;
//Nested for loops used to iterate through each pixel
for (int y = 0; y < bmp.Height; y++)
{
//Adjust the progress bar
progressBar1.Value = y + 1;
for (int x = 0; x < bmp.Width; x++)
{
//Get the color of each pixel
Color argb = bmp.GetPixel(x, y);
//Generate and add a random component to each value of RGB
R = argb.R + rnd.Next(-trackbar.Value,trackbar.Value);
G = argb.G + rnd.Next(-trackbar.Value,trackbar.Value);
B = argb.B + rnd.Next(-trackbar.Value,trackbar.Value);
//Ensure the R value is within bounds
if (R > 255)
{
R = 255;
}
if (R < 0)
{
R = 0;
}
//Ensure the G value is within bounds
if (G > 255)
{
G = 255;
}
if (G < 0)
{
G = 0;
}
//Ensure the B value is within bounds
if (B > 255)
{
B = 255;
}
if (B < 0)
{
B = 0;
}
//Change the color of the pixel to its new value
argb = Color.FromArgb(argb.A, R, G, ;
//Apply the new color to the pixel
bmp.SetPixel(x, y, argb);
}
}
}
//Load the bmp to the picture box again
pbox.Image = bmp;
}
//When the contrast radio is checked
private **** contb_CheckedChanged(object sender, EventArgs e)
{
//Set the trackbar and labels to the proper values
trackbar.Maximum = 100;
trackbar.Value = 0;
label1.Text = trackbar.Value.ToString();
label2.Text = "";
label3.Text = "Less";
label4.Text = "More";
}
//When then tint radio is checked
private **** tintb_CheckedChanged(object sender, EventArgs e)
{
//Set the trackbar and labels to the proper values
trackbar.Maximum = 100;
trackbar.Value = 50;
label3.Text = "Green";
label4.Text = "Red";
}
//When the B&W radio is checked
private **** bwb_CheckedChanged(object sender, EventArgs e)
{
//Set the trackbar and labels to the proper values
trackbar.Maximum = 100;
trackbar.Value = 0;
label1.Text = trackbar.Value.ToString();
label2.Text = "";
label3.Text = "Less";
label4.Text = "More";
}
//When the noise radio is checked
private **** nb_CheckedChanged(object sender, EventArgs e)
{
//Set the trackbar and labels to the proper values
trackbar.Maximum = 100;
trackbar.Value = 0;
label1.Text = trackbar.Value.ToString();
label2.Text = "";
label3.Text = "Less";
label4.Text = "More";
}
//When the trackbar value changes
private **** trackbar_Scroll(object sender, EventArgs e)
{
//If any of the three radios are checked do this
if (contb.Checked || bwb.Checked || nb.Checked)
{
//Set the trackbar label to the value of the bar
label1.Text = trackbar.Value.ToString();
//Set label2 blank
label2.Text = "";
}
if (tintb.Checked)
{
//When the value is above 50
if (trackbar.Value >= 50)
{
//Make the label say Green
label2.Text = "Green";
//Set the value of the trackbar label to a positive integer based on its distance from 50
label1.Text = (trackbar.Value - 50).ToString();
}
if (trackbar.Value < 50)
{
//Make the label say Red
label2.Text = "Red";
//Set the value of the trackbar label to a positive integer based on its distance from 50
label1.Text = (50 - trackbar.Value).ToString();
}
}
}
}
}
Türkçe;
Kod:
Bu C# ile yazılmış basit bir fotoğraf manipülasyonu programıdır. -- PhotoShop Tacky Edition
TurkHackTeam Dil Takımı
namespace ***Redacted****
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
//Eğer Load butonuna tıklandıysa
private **** loadb_Click(object sender, EventArgs e)
{
//Dosya diyaloğunu aç ve kullanıcının resim seçmesine izin ver
if (openFileDialog1.ShowDialog() == DialogResult.OK)
{
//Seçilen dosyayı picture box içine resim olarak yerleştirmeyi dene
try
{
pbox.Image******** = openFileDialog1.FileName;
//Eğer çalışıyorsa Transform butonunu aktif et
tb.Enabled = true;
}
//Resim özellikleri yanlışsa transform butonuna tıklanmasını engelle
{
tb.Enabled = false;
}
}
}
//Eğer transform butonuna tıklandıysa
private **** tb_Click(object sender, EventArgs e)
{
//Resimi picture boxun içine bitmap olarak yükle
Bitmap bmp = new Bitmap(pbox.Image);
//Progress bar büyüklüğünü belirleyin
progressBar1.Maximum = bmp.Height;
//Kontrast radio butonu seçiliyse,
if (contb.Checked)
{
// Her pikselin koordinatı elde etmek için iç içe döngüler kullanın
for (int y = 0; y < bmp.Height; y++)
{
progressBar1.Value = y+1;
for (int x = 0; x < bmp.Width; x++)
{
//Pixelin rengini al
Color argb = bmp.GetPixel(x, y);
int R;
int G;
int B;
//Değerin 128 den büyük olup olmadığını kontrol edin.
if (argb.R > 128)
{
//Değerin 255i geçmediğine emin olun
if (argb.R + (trackbar.Value / 5) > 255)
{
R = 255;
}
else
{
//R değerini daha yüksek bi değerle değiştirin
R = argb.R + (trackbar.Value / 5);
}
}
else
{
//Değerin 0ın altına düşmediğine emin olun
if (argb.R - (trackbar.Value / 5) < 0)
{
R = 0;
}
else
{
//R değerini daha düşük bir değerle değiştirin
R = argb.R - (trackbar.Value / 5);
}
}
//Yeşil için tekrar edin
if (argb.G > 128)
{
if (argb.G + (trackbar.Value / 5) > 255)
{
G = 255;
}
else
{
G = argb.G + (trackbar.Value / 5);
}
}
else
{
if (argb.G - (trackbar.Value / 5) < 0)
{
G = 0;
}
else
{
G = argb.G - (trackbar.Value / 5);
}
}
//Mavi için tekrar edin
if (argb.B > 128)
{
if (argb.B + (trackbar.Value / 5) > 255)
{
B = 255;
}
else
{
B = argb.B + (trackbar.Value / 5);
}
}
else
{
if (argb.B - (trackbar.Value / 5) < 0)
{
B = 0;
}
else
{
B = argb.B - (trackbar.Value / 5);
}
}
//Pixellerin renklerini yenileri ile değiştirin
argb = Color.FromArgb(argb.A, R, G, ;
//Yeni pixellerin renklerini uygulayın
bmp.SetPixel(x, y, argb);
}
}
}
//Eğer tint radio butonu seçiliyse
if (tintb.Checked)
{
//Yeşil için bir integer değeri
int G;
//Mavi için bir integer değeri
int R;
// Her pikselin koordinatı elde etmek için iç içe döngüler kullanın
for (int y = 0; y < bmp.Height; y++)
{
//Progress bar ın süre ile ayarlanması
progressBar1.Value = y + 1;
for (int x = 0; x < bmp.Width; x++)
{
//Geçerli pixelin rengini elde edin
Color argb = bmp.GetPixel(x, y);
//Değerleri 0 a getirin
R = 0;
G = 0;
//Kırmızı yada yeşil, hangisine tint edeceğimizi belirleyin
if (trackbar.Value >= 50)
{
//R yi uygun değere ayarlayın
R = argb.R;
// Ayarlarken Gnin değer sınırları içinde kaldığından emin olun
if (((trackbar.Value - 50) + argb.G) > 255)
{
G = 255;
}
else
{
//Yeşili ne kadar tint etmek istediğinize göre ayarlayın
G = argb.G + (trackbar.Value - 50);
}
}
if (trackbar.Value < 50)
{
//G yi uygun değere ayarlayın
G = argb.G;
// Ayarlarken Rnin değer sınırları içinde kaldığından emin olun
if ((50 - trackbar.Value + argb.R) > 255)
{
R = 255;
}
else
{
//Kırmızı değerini ne kadar tint yapmak istediğinize göre ayarlayın
R = argb.R + (50 - trackbar.Value);
}
}
//Pixel renklerini yeni renkler ile değiştirin
argb = Color.FromArgb(argb.A, R, G, argb.;
//Pixellerin yeni renklerini onaylayın
bmp.SetPixel(x, y, argb);
}
}
}
//Siyah/Beyaz radio butonu işaretliyse
if (bwb.Checked)
{
//RGB için double değişkeni kullanılır;
//İnteger değerleri hesaplamaların sonunda RGB ye atanmak için kullanılır
int Ri;
int Gi;
int Bi;
//Hesaplamalarda double değişkeni kullanıldığından sayıları yuvarlayamayız
double R;
double G;
double B;
//Yüzde kaç grileştirme seçtik
double p = (double)trackbar.Value / 100;
// Her pikselin koordinatı elde etmek için iç içe döngüler kullanın for (int y = 0; y < bmp.Height; y++)
{
//Progress Barı ekleyin
progressBar1.Value = y + 1;
for (int x = 0; x < bmp.Width; x++)
{
//Her pikselin renk değerini elde edin
Color argb = bmp.GetPixel(x, y);
// İlgili double değerler atayın
R = argb.R;
G = argb.G;
B = argb.B;
//Ortalamayı belirleyin
ave = (R + G + / 3;
//Hesaplanmış siyah ve beyaz değerlerini integer olarak biçimlendir
Ri = (int)(R + ((ave - R) * p));
Gi = (int)(G + ((ave - G) * p));
Bi = (int)(B + ((ave - * p));
//Pixellerin renklerini yeni değerler ile değiştir
argb = Color.FromArgb(argb.A, Ri, Gi, Bi);
//Pixellerin yeni renklerini onayla
bmp.SetPixel(x, y, argb);
}
}
}
//Noise radio butonu seçiliyse
if (nb.Checked)
{
//Noise oluşturmak için rastgele sayı
Random rnd = new Random();
//Kırmızı değeri
int R;
//Yeşil değeri
int G;
//Mavi değeri
int B;
// Her pikselin koordinatı elde etmek için iç içe döngüler kullanın
for (int y = 0; y < bmp.Height; y++)
{
//Progress bar ı ayarlayın
progressBar1.Value = y + 1;
for (int x = 0; x < bmp.Width; x++)
{
//Her pixelin rengini alın
Color argb = bmp.GetPixel(x, y);
// Oluşturun ve RGB her değerine rastgele bir bileşen ekleyin
R = argb.R + rnd.Next(-trackbar.Value,trackbar.Value);
G = argb.G + rnd.Next(-trackbar.Value,trackbar.Value);
B = argb.B + rnd.Next(-trackbar.Value,trackbar.Value);
//R değerininin sınırlar içinde olduğuna emin olun
if (R > 255)
{
R = 255;
}
if (R < 0)
{
R = 0;
}
//G değerininin sınırlar içinde olduğuna emin olun
if (G > 255)
{
G = 255;
}
if (G < 0)
{
G = 0;
}
//B değerinin sınırlar içinde olduğuna emin olun
if (B > 255)
{
B = 255;
}
if (B < 0)
{
B = 0;
}
//Pixellerin rengini yenileriyle değiştirin
argb = Color.FromArgb(argb.A, R, G, ;
//Pixellerin yeni rengini onaylayın
bmp.SetPixel(x, y, argb);
}
}
}
//Picture Boxa bmp yi tekrar yükleyin
pbox.Image = bmp;
}
//Kontrast radio butonu seçiliyken
private **** contb_CheckedChanged(object sender, EventArgs e)
{
// Doğru değerlere trackbar ve etiketleri ayarlayın
trackbar.Maximum = 100;
trackbar.Value = 0;
label1.Text = trackbar.Value.ToString();
label2.Text = "";
label3.Text = "Less";
label4.Text = "More";
}
//Tint radio butonu seçiliyken
private **** tintb_CheckedChanged(object sender, EventArgs e)
{
// Doğru değerlere trackbar ve etiketleri ayarlayın
trackbar.Maximum = 100;
trackbar.Value = 50;
label3.Text = "Green";
label4.Text = "Red";
}
//Siyah beyaz radio butonu seçiliyken
private **** bwb_CheckedChanged(object sender, EventArgs e)
{
// Doğru değerlere trackbar ve etiketleri ayarlayın
trackbar.Maximum = 100;
trackbar.Value = 0;
label1.Text = trackbar.Value.ToString();
label2.Text = "";
label3.Text = "Less";
label4.Text = "More";
}
//Noise radio butonu seçiliyken
private **** nb_CheckedChanged(object sender, EventArgs e)
{
// Doğru değerlere trackbar ve etiketleri ayarlayın
trackbar.Maximum = 100;
trackbar.Value = 0;
label1.Text = trackbar.Value.ToString();
label2.Text = "";
label3.Text = "Less";
label4.Text = "More";
}
//Trackbar değerleri değiştiğinde
private **** trackbar_Scroll(object sender, EventArgs e)
{
//Eğer 3 radio butonundan biri seçiliyse bunu yap
if (contb.Checked || bwb.Checked || nb.Checked)
{
// Bar değerine trackbar etiket ayarla label1.Text = trackbar.Value.ToString();
//Label 2 yi boş olarak ayarla
label2.Text = "";
}
if (tintb.Checked)
{
//Değer 50 yi geçtiğinde
if (trackbar.Value >= 50)
{
//Label yeşil göstersin
label2.Text = "Green";
label1.Text = (trackbar.Value - 50).ToString();
}
if (trackbar.Value < 50)
{
//Label kırmızı desin
label2.Text = "Red";
label1.Text = (50 - trackbar.Value).ToString();
}
}
}
}
}
Kodlar tarafımdan yazılmamış, sadece programın çalışması bozulmayacak şekilde Türkçeye çevrilebilecek yerler çevrilmiştir.
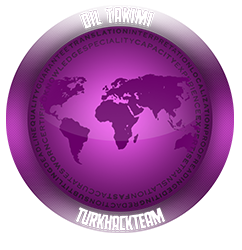